E-commerce Website
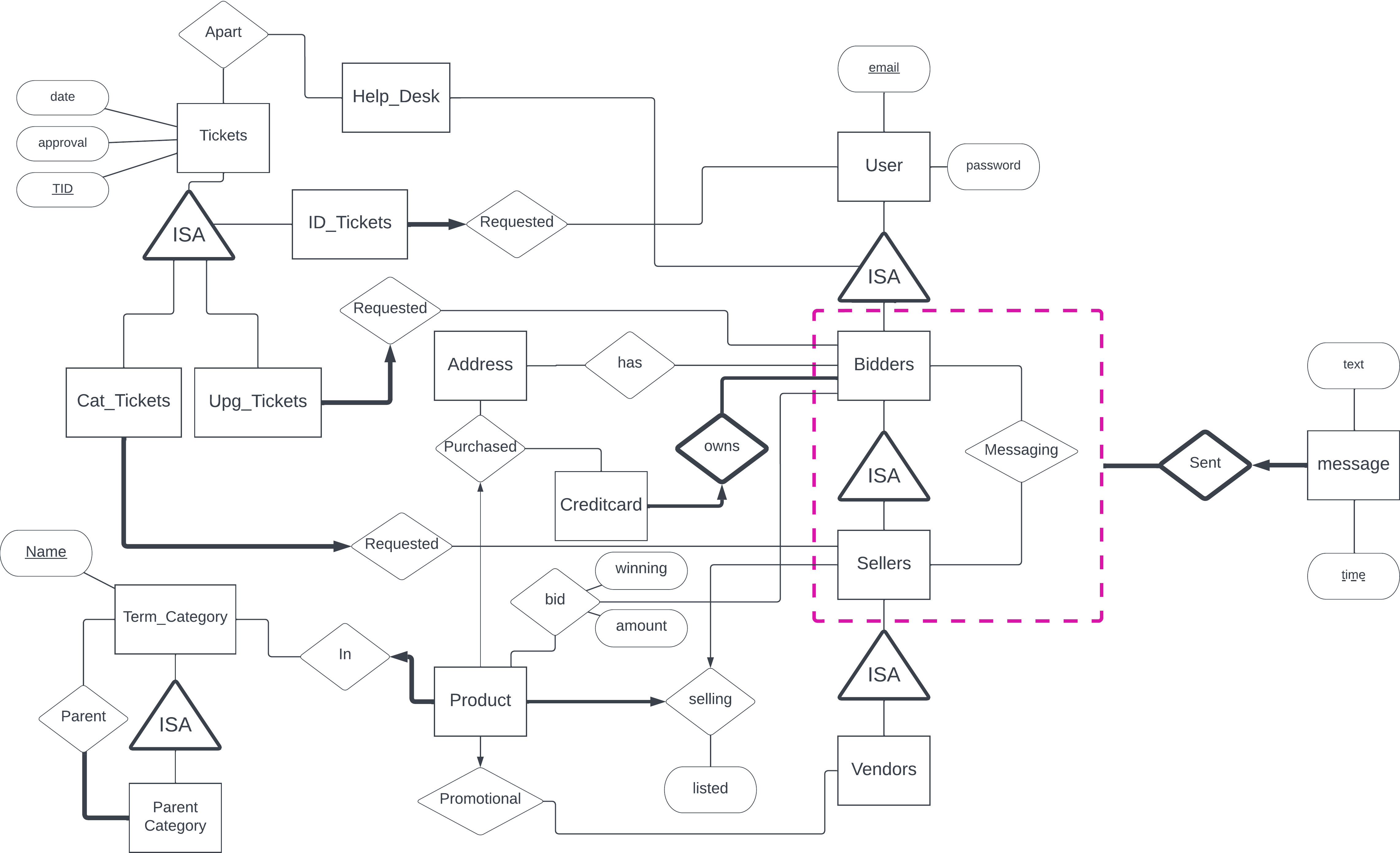
Introduction
The E-Commerece website was developed for a database management class in university for a fictitious customer that wanted an auction similar website for the local community. The development process was divided into two parts. First off, the goal was to create a feasibility study for the website which will outline how the website will be made from the code to the database design. The second stage was developing the website itself.
Deploying LionAuction: A Feasibility Study
Here's a summary of the results of the study.
Introduction
The LionAuction project is designed to create an online auction platform specifically for the LSU community. Modeled after successful platforms like eBay, LionAuction aims to facilitate the buying and selling of used and new items, targeting a niche audience within LSU.
Background
The idea for LionAuction emerged from a market investigation at LSU, which identified a need for a local platform where students and staff could easily auction items, particularly textbooks. With a significant portion of college students sourcing their textbooks second-hand, this platform addresses a clear demand.
Project Scope
The scope of this feasibility study includes the design and implementation strategies for the LionAuction platform, focusing on the database design and technical considerations. However, aspects such as costs, data hosting, and promotional strategies were not covered.
Requirement Analysis
The project identified several user roles, including bidders, sellers, and help desk employees, each with distinct functionalities on the platform. The website is designed to be user-friendly, featuring registration, product listing, bidding, and customer support.
Database Design
A significant portion of the project was dedicated to designing a robust database. The conceptual database design includes an entity-relationship (ER) model that outlines the relationships between different entities like users, products, bids, and tickets. This model ensures that the platform can handle the complex interactions between various users and items efficiently.
Technology Survey
The project compared different technologies for implementation, such as PHP vs. Python for the backend and MySQL vs. SQLite for database management. After a thorough evaluation, recommendations were made to use technologies that align with industry standards and best practices.
Conclusion
The study concludes that developing the LionAuction platform is feasible from a technical standpoint. The proposed database design and technology choices provide a solid foundation for the platform's development. However, further analysis is required to address other aspects like cost, server infrastructure, and regulatory compliance.
Next Steps
Moving forward, the project will need to consider additional factors such as funding, regulatory requirements, and a detailed implementation plan. These will be crucial for turning the LionAuction concept into a fully functional platform.
Building the LionAuction Website: A Developer's Journey
Technology Stack
The website was developed using a combination of Flask for the backend, SQLite for the database, and basic HTML/CSS for the frontend. Flask was chosen for its simplicity and flexibility, making it ideal for this project. SQLite was selected as the database due to its lightweight nature, which is sufficient for the platform’s current needs.
Backend Development
The backend is the heart of LionAuction, managing user sessions, handling requests, and interacting with the database. Key functionalities were implemented in the app.py
file, which serves as the entry point for the Flask application.
User Authentication and Session Management
The website supports multiple user roles, including bidders, sellers, and help desk employees. Session management was crucial for ensuring that users are correctly routed based on their roles. Upon logging in, users are redirected to the appropriate dashboard based on their role, with session data securely managed using Flask's session handling capabilities.@app.route('/') def index(): if 'email' not in session.keys(): return redirect('/login') if 'user_type' not in session.keys(): return redirect('/select_user_type') # Redirect based on user type if session['user_type'] == 'seller': return redirect('/seller') elif session['user_type'] == 'bidder': return redirect('/bidder') elif session['user_type'] == 'helpdesk': return redirect('/helpdesk')
Database Interactions
All interactions with the SQLite database are managed through a dedicated module (dbManager.py
). This module handles everything from inserting new users and listings to retrieving auction details and processing bids. The use of parameterized queries ensures that the database operations are secure and efficient.def insert_user(email, password): hashPassword = hashlib.md5(password.encode('utf-8')).hexdigest() connection = sql.connect('database.db') connection.execute('INSERT INTO Users (email, password) VALUES (?,?);', (email, hashPassword)) connection.commit()
Frontend Development
The frontend of LionAuction was designed to be straightforward and intuitive, focusing on the core functionalities of listing items, placing bids, and managing user accounts. Templates were created using Flask's rendering engine, allowing for dynamic content to be displayed based on the user's actions.
Responsive Design
The platform includes multiple pages, such as the main bidding page, seller listings, and user profiles, all designed to be responsive and accessible on various devices. For example, the bidder’s homepage dynamically displays current auction listings based on the user’s search criteria.@app.route('/bidder', methods=['POST', 'GET']) def bidder(): value = dbm.get_categories() return render_template('listing.html', listings=dbm.get_auction_listings(), title="Home", rtn=value, home=True)
Form Handling
Form submissions are an integral part of the website, whether it's logging in, creating a new auction, or placing a bid. Each form is processed on the server side, with appropriate validation and error handling to ensure smooth user interactions.@app.route('/create_user', methods=['POST', 'GET']) def create_user(): if request.method == 'POST': email = request.form['email'] password = request.form['password'] if dbm.is_user(email): return render_template('create_user.html', msg="Account with this email already exists") dbm.insert_user(email, password) return redirect('/login') return render_template('create_user.html')
REST API Integration
As the project evolved, it became necessary to implement a REST API for handling requests more efficiently. This API was designed to support various operations such as retrieving auction listings, placing bids, and managing user profiles. Here's an example of a REST API endpoint that retrieves auction listings based on user input:
@app.route('/api/auctions', methods=['GET'])
def get_auctions():
search_input = request.args.get('search')
listings = dbm.search_auction_listings(search_input)
return jsonify(listings)
This API endpoint accepts GET requests with search parameters and returns a JSON response containing the relevant auction listings. The integration of REST APIs has enhanced the flexibility of the platform, enabling easier communication between the frontend and backend.
Challenges and Solutions
One of the primary challenges was ensuring the secure handling of user data, particularly during authentication and session management. This was addressed by using Flask's built-in session management along with hashing techniques for storing passwords securely in the database.
Another challenge was creating a scalable database design that could efficiently handle various user actions, from creating listings to placing bids. The database schema was carefully designed to manage these operations effectively, with indexing and query optimization techniques employed to enhance performance.
Conclusion
Building the LionAuction website was a complex yet rewarding experience. The project provided valuable insights into full-stack development, particularly in the context of creating a specialized auction platform. With the foundational elements in place, future iterations of the website can focus on scaling, additional features, and enhanced user experience.